Image Filter Directive
The v-image-filter
directive is used to apply filters to images.
Usage

Hover
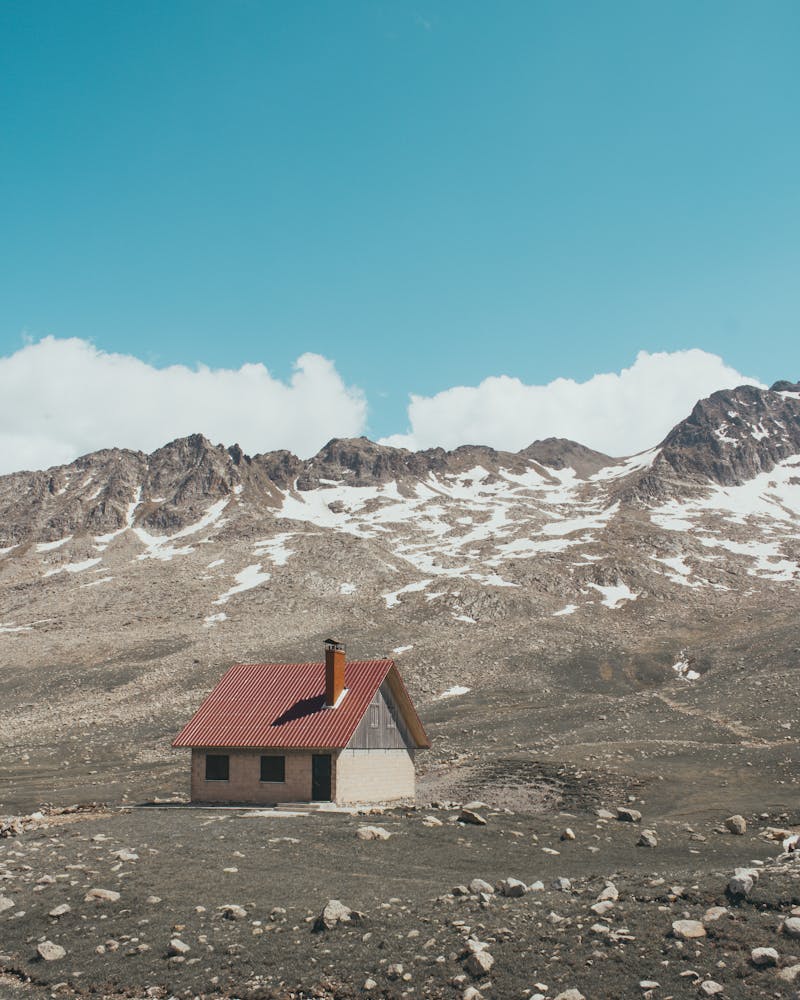
Hover
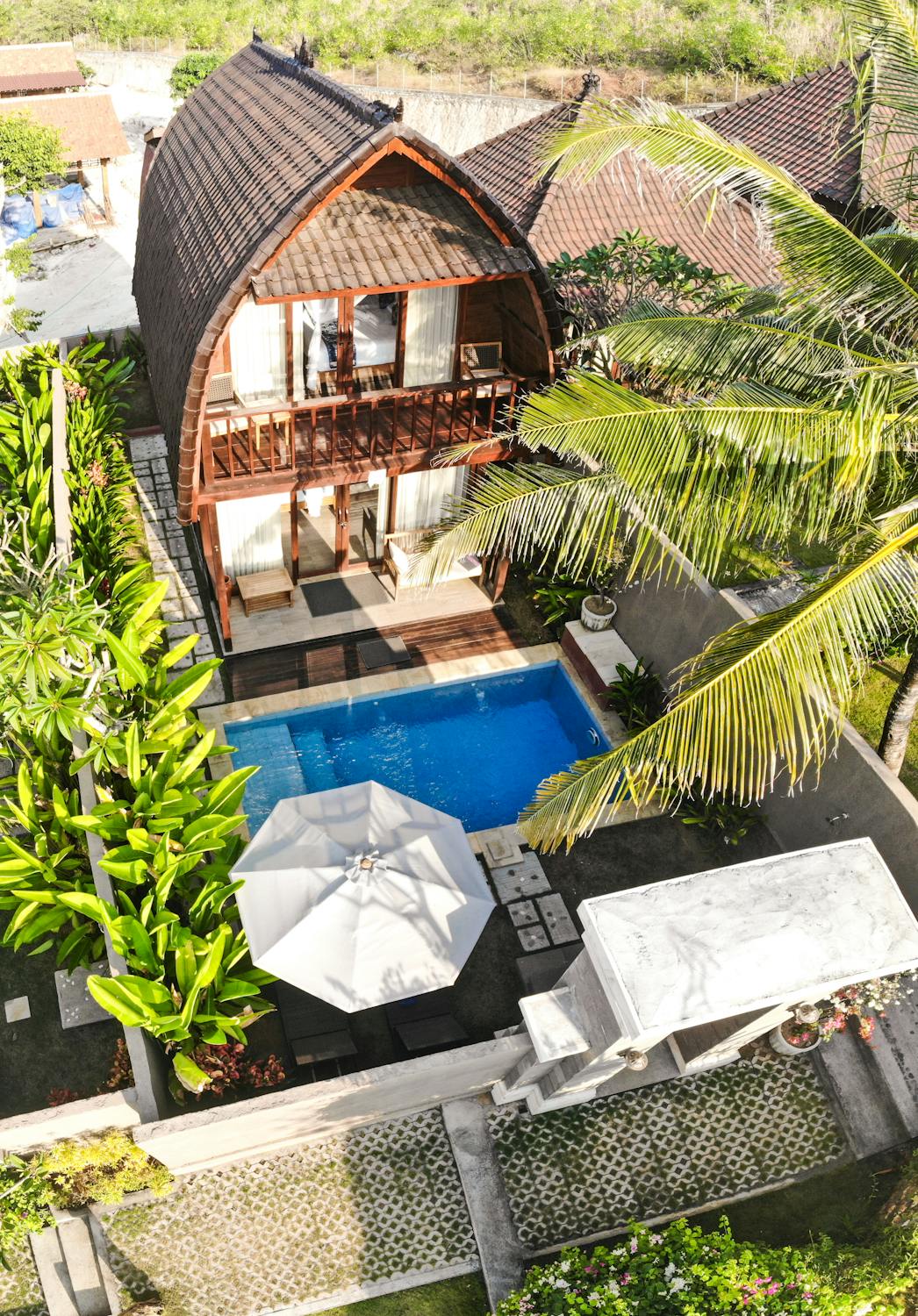
Click(Dbl Cick to remove)
View Code
vue
<template>
<div
class="flex justify-start flex-col md:flex-row space-y-2 md:space-y-0 md:space-x-2 py-5"
>
<div v-for="(item, index) in images" :key="index">
<img
v-image-filter:[item.arg]="{
filter: item.filter,
value: item.value,
}"
:src="item.src"
class="w-64 h-64 object-cover rounded-xl shadow-xl"
/>
<p class="text-xs">
{{ item.arg === "hover" ? "Hover" : "Click(Dbl Cick to remove)" }}
</p>
</div>
</div>
</template>
<script setup>
import { ref } from "vue";
const images = ref([
{
src: "https://images.pexels.com/photos/2980955/pexels-photo-2980955.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=2",
filter: "blur",
value: "1",
arg: "hover",
},
{
src: "https://images.pexels.com/photos/2189666/pexels-photo-2189666.jpeg?auto=compress&cs=tinysrgb&w=800",
filter: "blur",
value: "3",
arg: "hover",
},
{
src: "https://images.pexels.com/photos/2480608/pexels-photo-2480608.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=2",
filter: "grayscale",
value: "100",
arg: "click",
},
]);
</script>
<style scoped></style>
Arguments
The v-image-filter
takes the following arguments:
Argument | Description |
---|---|
hover | The filter will be applied on hover. |
click | The filter will be applied on click. |
Modifiers
The v-image-filter
takes the following modifiers:
Modifier | Description |
---|---|
blur | The image will be blurred. |
brightness | The image will be brightened. |
contrast | The image will be contrasted. |
grayscale | The image will be grayscaled. |
hue-rotate | The image will be hue-rotated. |
invert | The image will be inverted. |
opacity | The image will be opacity-adjusted. |
saturate | The image will be saturated. |
sepia | The image will be sepia-toned. |
drop-shadow | The image will have a drop shadow. |
Source Code
js
export const vImageFilter = {
mounted(el, binding) {
const { filter, value, arg } = binding.value;
const applyFilters = () => {
console.log("applyFilters");
el.classList.add(filter);
el.style.filter = `
${filter === "grayscale" ? `grayscale(${value || 100}%) ` : ""}
${filter === "blur" ? `blur(${value || 0}px) ` : ""}
${filter === "brightness" ? `brightness(${value || 100}%) ` : ""}
${filter === "contrast" ? `contrast(${value || 100}%) ` : ""}
${filter === "invert" ? "invert(100%) " : ""}
${filter === "opacity" ? `opacity(${value || 1}) ` : ""}
${filter === "saturate" ? `saturate(${value || 100}%) ` : ""}
${filter === "sepia" ? `sepia(${value || 0}%) ` : ""}
${filter === "hueRotate" ? `hue-rotate(${value || 0}deg) ` : ""}
${
filter === "dropShadow"
? `drop-shadow(${value || "2px 2px 2px rgba(0, 0, 0, 0.5)"})`
: ""
}
`;
};
const resetFilters = () => {
el.style.filter = "none";
};
const onHover = binding.arg === "hover";
const onClick = binding.arg === "click";
console.log("onHover", onHover);
if (onHover) {
el.addEventListener("mouseenter", applyFilters);
el.addEventListener("mouseleave", resetFilters);
} else if (onClick) {
el.addEventListener("click", applyFilters);
el.addEventListener("dblclick", resetFilters);
}
},
};